Bài trước, chúng ta đã tìm hiểu một đặc điểm thú vị của HTML5: hỗ trợ vẽ các đối tượng 2D thông qua thẻ canvas. Hôm nay, chúng ta lại tiếp cận một API mới của HTML5, lưu trữ dữ liệu web ở phía máy khách.
Lưu trữ web là một đặc điểm kỹ thuật được đưa ra bởi W3C, hỗ trợ việc lưu trữ dữ liệu tại phiên làm việc của người dùng hoặc lưu trữ trong thời gian lâu dài. Nó cho phép lưu trữ 5 MB thông tin cho mỗi tên miền.
Có 2 loại:
- sesionStorage: lưu trữ dữ liệu trong một phiên làm việc (sẽ bị xóa khi đóng cửa sổ trình duyệt)
- localStorage: lưu trữ cục bộ (thông tin được lưu trữ lâu dài, không giới hạn thời gian)
Trước khi sử dụng sessionStorage và localStorage, chúng ta phải kiểm tra trình duyệt hiện tại có hỗ trợ 2 dạng lưu trữ này không?
Code tham khảo kiểm tra trình duyệt:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
| <! DOCTYPE HTML> < html > < head > < meta charset = "utf-8" > < title >Kiểm tra lưu trữ phiên</ title > < script > function checkSupport() { if (('sessionStorage' in window) && window['sessionStorage'] !== null) { alert("Trình duyệt hỗ trợ lưu trữ web"); return; } alert("Trình duyệt không hỗ trợ lưu trữ web"); } </ script > </ head > < body onLoad = "checkSupport()" > </ body > </ html > |
1
| if (('sessionStorage' in window) && window['sessionStorage'] !== null) |
1
| if (('localStorage' in window) && window['localStorage'] !== null) |
Có 3 cách để lưu trữ:
- Thông qua các hàm hỗ trợ sẵn: lưu trữ dạng key/value (biến/giá trị)
+ Gán giá trị cho biến: sessionStorage.setItem(“key”) = value (key,value có thể là chuỗi hoặc số)
sessionStorage.setItem(‘name’, ‘user’)
+ Lấy giá trị của biến: sessionStorage.getItem(“key”)
sessionStorage.getItem(‘name’)
+ Xóa một giá trị biến đã tạo: sessionStorage.removeItem(“key”)
sessionStorage.removeItem(‘name’)
+ Xóa tất cả các biến đã tạo: sessionStorage.clear() - Thông qua cú pháp mảng: sessionStorage[“key”] = value
sessionStorage[‘name’] = ‘user’ - Thông qua toán tử dấu chấm: sessionStorage.key = value
sessionStorage.name = “user”
Ví dụ đơn giản để lưu trữ dữ liệu:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
| <! DOCTYPE HTML> < html > < head > < meta charset = "utf-8" > < title >Working with Session Storage</ title > < script > function testStorage() { if(('sessionStorage' in window) && window['sessionStorage'] != null){ sessionStorage.setItem('name', 'Sarah'); document.write("The name is:" + sessionStorage.getItem('name') + " "); sessionStorage.setItem(6, 'Six'); document.write("The number 6 is : " + sessionStorage.getItem(6) + " "); sessionStorage["age"] = 20; document.write("The age is : " + sessionStorage.age); } } </ script > </ head > < body onLoad = "testStorage()" > </ body > </ html > |
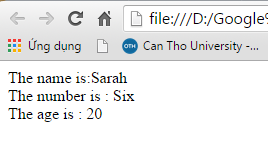
2. Ví dụ: Sử dụng sessionStorage để tạo phiên làm việc khi người dùng đăng nhập:
Kịch bản xử lý như sau:
- Tạo form đăng nhập.
- Tạo một trang thanhcong.html để hiển thị thông báo đăng nhập thành công.
- Nếu người dùng chưa đăng nhập -> hiển thị trang thanhcong.html thì có thông báo “Bạn chưa đăng nhập”
- Nếu người dùng vào trang đăng nhập rồi tiến hành đăng nhập -> sau khi đăng nhập thành công, qua trang thanhcong.html và hiển thị “Chúc mừng bạn tên đăng nhập đã đăng nhập thành công”.
Trang đăng nhập (dangnhap.html)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
| <! DOCTYPE HTML> < html > < head > < meta charset = "utf-8" > < title >Phiên làm việc người dùng</ title > < script > function kiemTra() { //kiem tra du lieu if(('sessionStorage' in window) && window['sessionStorage'] !== null){ // bỏ qua bước bắt lỗi tài khoản, mật khẩu // tạo sessionStorage với key là user lưu tài khoản người dùng sessionStorage.setItem('user', document.frm.txtTK.value); // tạo sessionStorage với key là pass lưu mật khẩu người dùng sessionStorage.setItem('pass', document.frm.txtMK.value); return true; }else alert("Không hỗ trợ"); } </ script > </ head > < body > < form name = "frm" onSubmit = "return kiemTra()" action = "thanhcong.html" > < table > < tr > < td >Tài khoản:</ td > < td >< input name = "txtTK" /></ td > </ tr > < tr > < td >Mật khẩu:</ td > < td >< input name = "txtMK" type = "password" /></ td > </ tr > < tr align = "center" > < td colspan = "2" >< input type = "submit" value = "Đăng nhập" /></ td > </ tr > </ table > </ form > </ body > </ html > |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
| <! doctype html> < html > < head > < meta charset = "utf-8" > < title >Thanh cong</ title > < script > if(sessionStorage.getItem('user') != null) document.write("Chúc mừng bạn " + sessionStorage.getItem('user') + " đã đăng nhập thành công"); else document.write('Bạn chưa đăng nhập'); /* Xử lý nút thoát : xóa biến lưu trữ user*/ function thoat(){ sessionStorage.removeItem('user'); location.href="thanhcong.html"; } </ script > </ head > < body > < form > < input value = "Thoát" type = "button" onClick = "thoat()" /> </ form > </ body > </ html > |
Mỗi lần người dùng vào trang web:
- Nếu là lần đầu tiên: khởi tạo biến localStorage[‘truy cập’] là 1.
- Nếu là lần thứ 2 về sau: cộng thêm 1 vào biến localStorage[‘truy cập’]
Code:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
function store() { if(('localStorage' in window) && window['localStorage'] != null){ if(localStorage.getItem('truycap') == null) localStorage.setItem('truycap', 1); else localStorage.setItem('truycap', parseInt(localStorage.getItem('truycap')) + 1); document.write("Số lần đăng nhập = " + localStorage.getItem('truycap')); } else{ alert("Trình duyệt không hỗ trợ LocalStorage"); } }
|
Kết quả:
Lần chạy đầu tiên:
Số lần đăng nhập = 1
Reload lại trang:
Số lần đăng nhập = 2
Các lần chạy tiếp theo -> mỗi lần tăng lên 1.
Tắt trình duyệt và chạy lại trình duyệt vừa rồi:
Số lần đăng nhập = 3
Các bạn có thể tham khảo thêm:
4. Ví dụ sử dụng localStorage để ghi nhớ thông tin đăng nhập:
Lần chạy đầu tiên:
Số lần đăng nhập = 1
Reload lại trang:
Số lần đăng nhập = 2
Các lần chạy tiếp theo -> mỗi lần tăng lên 1.
Tắt trình duyệt và chạy lại trình duyệt vừa rồi:
Số lần đăng nhập = 3
Các bạn có thể tham khảo thêm:
4. Ví dụ sử dụng localStorage để ghi nhớ thông tin đăng nhập:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
| <! DOCTYPE HTML> < html > < head > < meta charset = "utf-8" > < title >Lưu trữ cực bộ</ title > < script > function loadData(){ if(('localStorage' in window) && window['localStorage'] != null && localStorage.getItem('ghinho') ){ document.frmDangNhap.txtDN.value = localStorage.getItem('tendangnhap'); document.frmDangNhap.txtMK.value = localStorage.getItem('matkhau'); } } function store() { var tendangnhap = document.getElementById("txtDN").value; var matkhau = document.getElementById("txtMK").value; var ghinho = document.getElementById("chkGhiNho"); if(('localStorage' in window) && window['localStorage'] != null){ localStorage.setItem('tendangnhap', tendangnhap); localStorage.setItem('matkhau', matkhau); alert("Tên người dùng : " + localStorage.getItem('tendangnhap') + ", Mật khẩu : " + localStorage.getItem('matkhau')); } else{ alert("Trình duyệt không hỗ trợ LocalStorage"); } //ghi nho thong tin if(ghinho.checked) { localStorage.setItem('ghinho', 1); } } </ script > </ head > < body onLoad = "loadData()" > < form method = "get" name = "frmDangNhap" > < table width = "50%" border = "1" align = "center" > < tr > < th colspan = "2" scope = "col" >Đăng nhập </ th > </ tr > < tr > < td >Tên đăng nhập: </ td > < td >< input type = "text" id = "txtDN" name = "txtDN" /></ td > </ tr > < tr > < td >Mật khẩu:</ td > < td >< input type = "text" id = "txtMK" name = "txtMK" /></ td > </ tr > < tr > < td ></ td > < td >< input type = "button" value = "Đăng nhập" onClick = "store();" /> < input type = "checkbox" id = "chkGhiNho" />Ghi nhớ thông tin</ td > </ tr > </ table > </ form > </ body > </ html > |
0 nhận xét:
Đăng nhận xét